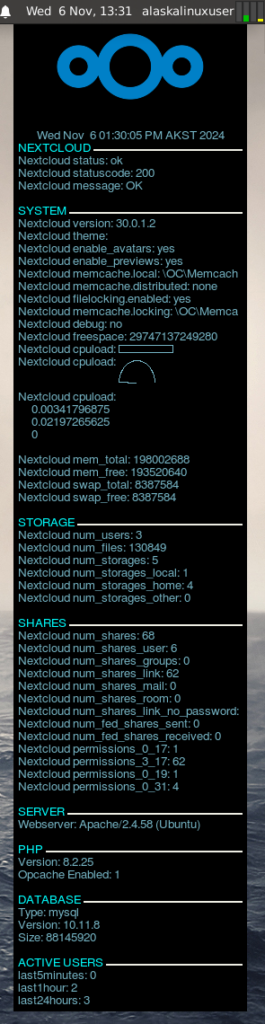
For any long time readers of this blog, you’ll know that I love to use Conky. It is a fun and useful desktop tool that can display the status of just about anything that you want, provided you can write a few scripts or bash commands. It has lots of built in status commands that just work out of the box, like CPU load, memory usage, and network statistics, but it also lets you run custom commands as well.
I also really like running my own NextCloud server. One problem though is that I don’t always know the status of my NC server without going to it directly to find out if everything is okay. Yesterday I decided to solve this with Conky.
There are three main parts to this setup. The first is creating a NextCloud token that you can use to download the NC status in an XML file. Fist you have to generate a token. I used openssl:
$ openssl rand -hex 32
This created a really long hex code. I then used that code in my NextCloud server folder to set the token:
$ sudo -u www-data php occ config:app:set serverinfo token --value REALLYLONGHEXCODEFROMPREVIOUSSTEP
Now I can download the NextCloud status with curl:
curl -H 'NC-Token: REALLYLONGHEXCODEFROMPREVIOUSSTEP' -X GET https://your.nextcloud.com/ocs/v2.php/apps/serverinfo/api/v1/info
Obviously, you have to replace the domain name with your server! But this will give you an XML file that has all of the status of your NC server! Now I just had to decide the best way to put that information into Conky. It would really slow Conky down to have to curl the file on every update, which for Conky can be as little as 1 second, or as long as you want. Fortunately, Conky has a way to call scripts only every so many seconds, while still updating the display whenever you want. It also allows you to create a separate thread to do work so you don’t slow down the updating of the display as well.
But first, I needed to write a script to get my NC server info with my new token. It looks like this:
#!/bin/bash
curl -H 'NC-Token: REALLYLONGHEXCODEFROMPREVIOUSSTEP' -X GET https://your.nextcloud.com/ocs/v2.php/apps/serverinfo/api/v1/info > /home/USERNAME/installed/scripts/nextcloudNew.info && cp /home/USERNAME/installed/scripts/nextcloudNew.info /home/USERNAME/installed/scripts/nextcloud.info && date > /home/USERNAME/installed/scripts/date.info
exit 0
This may look convoluted, but I assure you there is madness in this method. Er, I mean method to this madness…. You see the double ampersand (&&) means: “If this command is successful, then do this next command.” So, first we use curl with our token to get the server information, which we save the information at nextcloudNew.info. If that is successful, then copy that new.info file over the nextcloud.info file. If that works, then also write the date to the date.info file. This way, when the script is run, it has to download the latest data, if it does, we update the info and date. If it doesn’t it will be the old info and date. That way, if I see the old time/date, I know it is no longer updating. Also, if you curl to the current file that Conky will use, I’ve had a delay due to slow internet causing Conky to display errors because not all of the data is there yet.
Now we need to call it and farm it all into Conky. The key part is these bash commands:
cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/mem_free'
This command will use xmlstarlet to select the text of the field with the value (name) of ocs -> data -> nextcloud -> system -> mem_free. So we simply call this command for each item in our XML file. And we do that in our Conky config file, which by default is .conkyrc in your home directory:
######################
## Conky settings ##
######################
update_interval 10
total_run_times 0
net_avg_samples 1
cpu_avg_samples 1
imlib_cache_size 0
double_buffer yes
no_buffers yes
#####################
## Text settings ##
#####################
use_xft yes
xftfont FreeSans:size=10
override_utf8_locale yes
text_buffer_size 2048
#############################
## Window specifications ##
#############################
own_window_class Conky
own_window yes
own_window_type normal
own_window_transparent yes
own_window_hints undecorated,below,sticky,skip_taskbar,skip_pager
alignment top_right
gap_x 25
gap_y 10
minimum_size 182 0
maximum_width 250
default_bar_size 60 8
#########################
## Graphics settings ##
#########################
draw_shades no
default_color FFFFFF
## Colors to use for the text. ##
## color0 Main headings. ##
## color1 Bars and graphics. ##
## color2 regular text. ##
color0 00FFFF
color1 eeeee4
color2 76b5c5
TEXT
${texeci 600 /home/USERNAME/installed/scripts/getnextclouddata.sh}
## Begin text of conky output. ##
## This picture needs to be at this location or it will not display.
${alignc}${image ~/installed/scripts/nextcloud.png -p 29,0 -s 192x86 -f 300}
${alignc}${color2}${exec cat /home/USERNAME/installed/scripts/date.info | tail -n1}
${color0}NEXTCLOUD ${color1}${hr 2}${color2}
Nextcloud status: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/meta/status'}
Nextcloud statuscode: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/meta/statuscode'}
Nextcloud message: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/meta/message'}
${color0}SYSTEM ${color1}${hr 2}${color2}
Nextcloud version: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/version'}
Nextcloud theme: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/theme'}
Nextcloud enable_avatars: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/enable_avatars'}
Nextcloud enable_previews: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/enable_previews'}
Nextcloud memcache.local: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/memcache.local'}
Nextcloud memcache.distributed: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/memcache.distributed'}
Nextcloud filelocking.enabled: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/filelocking.enabled'}
Nextcloud memcache.locking: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/memcache.locking'}
Nextcloud debug: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/debug'}
Nextcloud freespace: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/freespace'}
# CPULOAD
### bar
Nextcloud cpuload: ${execbar cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/cpuload/element'}
### gauge
Nextcloud cpuload: ${execgauge cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/cpuload/element'}
### text
Nextcloud cpuload: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/cpuload'}
# CPULOAD END
# MEMORY
Nextcloud mem_total: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/mem_total'}
Nextcloud mem_free: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/mem_free'}
Nextcloud swap_total: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/swap_total'}
Nextcloud swap_free: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/system/swap_free'}
# MEMORY END
${color0}STORAGE ${color1}${hr 2}${color2}
Nextcloud num_users: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/storage/num_users'}
Nextcloud num_files: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/storage/num_files'}
Nextcloud num_storages: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/storage/num_storages'}
Nextcloud num_storages_local: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/storage/num_storages_local'}
Nextcloud num_storages_home: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/storage/num_storages_home'}
Nextcloud num_storages_other: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/storage/num_storages_other'}
${color0}SHARES ${color1}${hr 2}${color2}
Nextcloud num_shares: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_shares'}
Nextcloud num_shares_user: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_shares_user'}
Nextcloud num_shares_groups: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_shares_groups'}
Nextcloud num_shares_link: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_shares_link'}
Nextcloud num_shares_mail: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_shares_mail'}
Nextcloud num_shares_room: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_shares_room'}
Nextcloud num_shares_link_no_password: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_shares_link_no_password'}
Nextcloud num_fed_shares_sent: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_fed_shares_sent'}
Nextcloud num_fed_shares_received: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/num_fed_shares_received'}
Nextcloud permissions_0_17: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/permissions_0_17'}
Nextcloud permissions_3_17: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/permissions_3_17'}
Nextcloud permissions_0_19: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/permissions_0_19'}
Nextcloud permissions_0_31: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/nextcloud/shares/permissions_0_31'}
${color0}SERVER ${color1}${hr 2}${color2}
Webserver: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/webserver'}
${color0}PHP ${color1}${hr 2}${color2}
Version: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/version'}
#Memory Limit: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/memory_limit'}
#Max Execution Time: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/max_execution_time'}
#Upload Max Filesize: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/upload_max_filesize'}
#Opcache Revalidate Freq: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/opcache_revalidate_freq'}
Opcache Enabled: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/opcache/opcache_enabled'}
#Opcache Memory Usage: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/opcache/memory_usage'}
#Opcache Interned Strings Usage: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/opcache/interned_strings_usage'}
#Opcache Statistics: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/opcache/opcache_statistics'}
#JIT Enabled: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/opcache/jit/enabled'}
#APCU Cache: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/apcu/cache'}
#APCU sma: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/apcu/sma'}
#Extensions: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/php/extensions'}
${color0}DATABASE ${color1}${hr 2}${color2}
Type: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/database/type'}
Version: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/database/version'}
Size: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/server/database/size'}
${color0}ACTIVE USERS ${color1}${hr 2}${color2}
last5minutes: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/activeUsers/last5minutes'}
last1hour: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/activeUsers/last1hour'}
last24hours: ${exec cat /home/USERNAME/installed/scripts/nextcloud.info | xmlstarlet sel -t -v '/ocs/data/activeUsers/last24hours'}
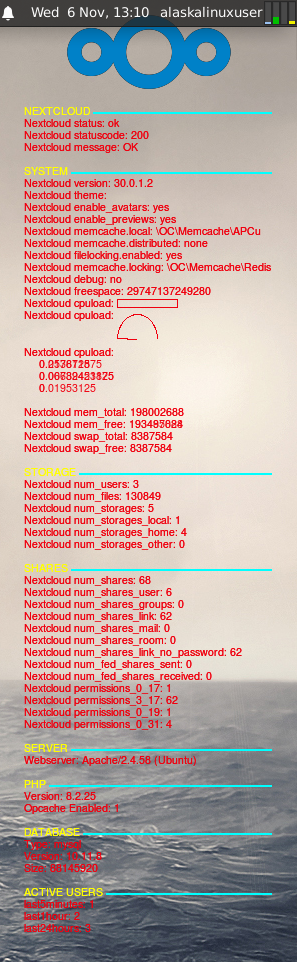
Noteworthy is that I only call the script to get the NC server status every 10 minutes. I do that as a separate thread:
${texeci 600 /home/USERNAME/installed/scripts/getnextclouddata.sh}
However, the Conky display updates every 10 seconds.
update_interval 10
You could easily change this to your needs, but what I find is sometimes when switching virtual desktops/spaces, the conky window will dissapear until it is updated again. Realistically, you could call this every 60 seconds or longer if you wanted to, since in my case the NC server info is only curled every 10 minutes. This parsing of the xml file doesn’t cause any system load on my machine, though, when I checked, with Conky running and me doing nothing else, the system load is still less than 4%.
You will also note in this file that I have several lines commented out. You can use this file and comment out any lines you don’t want to see, and uncomment other lines if you do wish to see them. I also displayed the CPU load using numbers, a bar, and a gauge just for the purpose of demonstration. You are welcome to comment out any of them if you wanted to use this file.
You could also make the background transparent, as I did in the second screenshot, but my laptop had some sort of issue with compositing that, and would often “mess up” different parts of the screen while displaying Conky transparently. The color scheme is also easily changed with the color declarations at the top:
## Colors to use for the text. ##
## color0 Main headings. ##
## color1 Bars and graphics. ##
## color2 regular text. ##
color0 00FFFF
color1 eeeee4
color2 76b5c5
Either way, if it is at all useful to you, you are welcome to borrow what I am using, or bounce off of it to make something better.
Linux – keep it simple.